In today’s tutorial, we are going to learn to do file transfer using a TCP socket in the python3 programming language. This is the most basic file transfer program that we can do using a client-server architecture. Here, we are going to do the build a client and a server program file, where the client read the data from a text file and send it to the server. The server receives it and saves the data to another text file.
The overall procedure for the TCP file transfer is presented in the figure below.
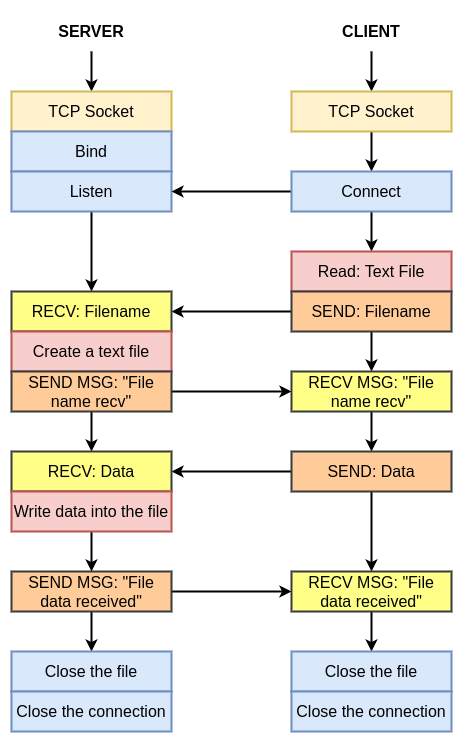
Related Tutorials
More Interested in File Transfer using the C programming language? Check out the tutorial below to learn more.
What is TCP?
TCP stands for Transmission Control Protocol. It is a communication protocol that is designed for end-to-end data transmission over a network. TCP is basically the ” standard” communication protocol for data transmission over the Internet.
It is a highly efficient and reliable communication protocol as it uses a three-way handshake to connect the client and the server. It is a process that requires both the client and the server to exchange synchronization (SYN) and acknowledge (ACK) packets before the data transfer takes place.
Some of the features of the TCP are as follows:
- It provides end-to-end communication.
- It is a connection-oriented protocol.
- It provides error-checking and recovery mechanisms.
Project Structure
The project is divided into two files:
- server.py
- client.py
The client.py contains the code, used to read a text file and send its data to the server. While the server.py file receives the data sent by the client and save it to a text file.
File Transfer: SERVER
The server performs the following functions:
- Create a TCP socket.
- Bind the IP address and PORT to the server socket.
- Listening for the clients.
- Accept the connection from the client.
- Receive the filename from the client and create a text file.
- Send a response back to the client.
- Receive the text data from the client.
- Write (save) the data into the text file.
- Send a response message back to the client.
- Close the text file.
- Close the connection.
import socket
IP = socket.gethostbyname(socket.gethostname())
PORT = 4455
ADDR = (IP, PORT)
SIZE = 1024
FORMAT = "utf-8"
def main():
print("[STARTING] Server is starting.")
""" Staring a TCP socket. """
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
""" Bind the IP and PORT to the server. """
server.bind(ADDR)
""" Server is listening, i.e., server is now waiting for the client to connected. """
server.listen()
print("[LISTENING] Server is listening.")
while True:
""" Server has accepted the connection from the client. """
conn, addr = server.accept()
print(f"[NEW CONNECTION] {addr} connected.")
""" Receiving the filename from the client. """
filename = conn.recv(SIZE).decode(FORMAT)
print(f"[RECV] Receiving the filename.")
file = open(filename, "w")
conn.send("Filename received.".encode(FORMAT))
""" Receiving the file data from the client. """
data = conn.recv(SIZE).decode(FORMAT)
print(f"[RECV] Receiving the file data.")
file.write(data)
conn.send("File data received".encode(FORMAT))
""" Closing the file. """
file.close()
""" Closing the connection from the client. """
conn.close()
print(f"[DISCONNECTED] {addr} disconnected.")
if __name__ == "__main__":
main()
FIle Transfer: CLIENT
The client performs the following functions:
- Create a TCP socket for the client.
- Connect to the server.
- Read the data from the text file.
- Send the filename to the server.
- Receive the response from the server.
- Send the text file data to the server.
- Receive the response from the server.
- Close the file.
- Close the connection.
import socket
IP = socket.gethostbyname(socket.gethostname())
PORT = 4455
ADDR = (IP, PORT)
FORMAT = "utf-8"
SIZE = 1024
def main():
""" Staring a TCP socket. """
client = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
""" Connecting to the server. """
client.connect(ADDR)
""" Opening and reading the file data. """
file = open("data/yt.txt", "r")
data = file.read()
""" Sending the filename to the server. """
client.send("yt.txt".encode(FORMAT))
msg = client.recv(SIZE).decode(FORMAT)
print(f"[SERVER]: {msg}")
""" Sending the file data to the server. """
client.send(data.encode(FORMAT))
msg = client.recv(SIZE).decode(FORMAT)
print(f"[SERVER]: {msg}")
""" Closing the file. """
file.close()
""" Closing the connection from the server. """
client.close()
if __name__ == "__main__":
main()
Summary
In this article, you have learned a simple file transfer using TCP in the python 3 programming language. Still, have some questions or queries? Just comment below. For more updates. Follow me.