File Transfer using TCP Socket in C
In this tutorial, you will learn how to perform a file (data) transfer over a TCP socket in the C programming language. You will see how a client reads the data from a text file sends it to the server and then saves the data back into a text file.
For this tutorial, I am going to use the TCP to establish the connection between the client and the server.
RELATED TUTORIALS:
What is TCP
TCP refers to the Transmission Control Protocol, which is a highly efficient and reliable protocol designed for end-to-end data transmission over an unreliable network.
A TCP connection uses a three-way handshake to connect the client and the server. It is a process that requires both the client and the server to exchange synchronization (SYN) and acknowledge (ACK) packets before the data transfer takes place.
Some important features of TCP:
- It’s a connection-oriented protocol.
- It provides error-checking and recovery mechanisms.
- It helps in end-to-end communication.
Project Structure
The project is divided into two files:
- client.c
- server.c
The client.c file contains the code for the client-side, which read the text file and sends it to the server and the server.c file receives the data from the client and saves it in a text file.
Client
The client performs the following functions.
- Start the program
- Declare the variables and structures required.
- A socket is created and the connect function is executed.
- The file is opened.
- The data from the file is read and sent to the server.
- The socket is closed.
- The program is stopped.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <arpa/inet.h>
#define SIZE 1024
void send_file(FILE *fp, int sockfd){
int n;
char data[SIZE] = {0};
while(fgets(data, SIZE, fp) != NULL) {
if (send(sockfd, data, sizeof(data), 0) == -1) {
perror("[-]Error in sending file.");
exit(1);
}
bzero(data, SIZE);
}
}
int main(){
char *ip = "127.0.0.1";
int port = 8080;
int e;
int sockfd;
struct sockaddr_in server_addr;
FILE *fp;
char *filename = "send.txt";
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if(sockfd < 0) {
perror("[-]Error in socket");
exit(1);
}
printf("[+]Server socket created successfully.\n");
server_addr.sin_family = AF_INET;
server_addr.sin_port = port;
server_addr.sin_addr.s_addr = inet_addr(ip);
e = connect(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
if(e == -1) {
perror("[-]Error in socket");
exit(1);
}
printf("[+]Connected to Server.\n");
fp = fopen(filename, "r");
if (fp == NULL) {
perror("[-]Error in reading file.");
exit(1);
}
send_file(fp, sockfd);
printf("[+]File data sent successfully.\n");
printf("[+]Closing the connection.\n");
close(sockfd);
return 0;
}
Server
The server performs the following functions.
- Start the program.
- Declare the variables and structures required.
- The socket is created using the socket function.
- The socket is binded to the specific port.
- Start listening for the connections.
- Accept the connection from the client.
- Create a new file.
- Receives the data from the client.
- Write the data into the file.
- The program is stopped.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <arpa/inet.h>
#define SIZE 1024
void write_file(int sockfd){
int n;
FILE *fp;
char *filename = "recv.txt";
char buffer[SIZE];
fp = fopen(filename, "w");
while (1) {
n = recv(sockfd, buffer, SIZE, 0);
if (n <= 0){
break;
return;
}
fprintf(fp, "%s", buffer);
bzero(buffer, SIZE);
}
return;
}
int main(){
char *ip = "127.0.0.1";
int port = 8080;
int e;
int sockfd, new_sock;
struct sockaddr_in server_addr, new_addr;
socklen_t addr_size;
char buffer[SIZE];
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if(sockfd < 0) {
perror("[-]Error in socket");
exit(1);
}
printf("[+]Server socket created successfully.\n");
server_addr.sin_family = AF_INET;
server_addr.sin_port = port;
server_addr.sin_addr.s_addr = inet_addr(ip);
e = bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
if(e < 0) {
perror("[-]Error in bind");
exit(1);
}
printf("[+]Binding successfull.\n");
if(listen(sockfd, 10) == 0){
printf("[+]Listening....\n");
}else{
perror("[-]Error in listening");
exit(1);
}
addr_size = sizeof(new_addr);
new_sock = accept(sockfd, (struct sockaddr*)&new_addr, &addr_size);
write_file(new_sock);
printf("[+]Data written in the file successfully.\n");
return 0;
}
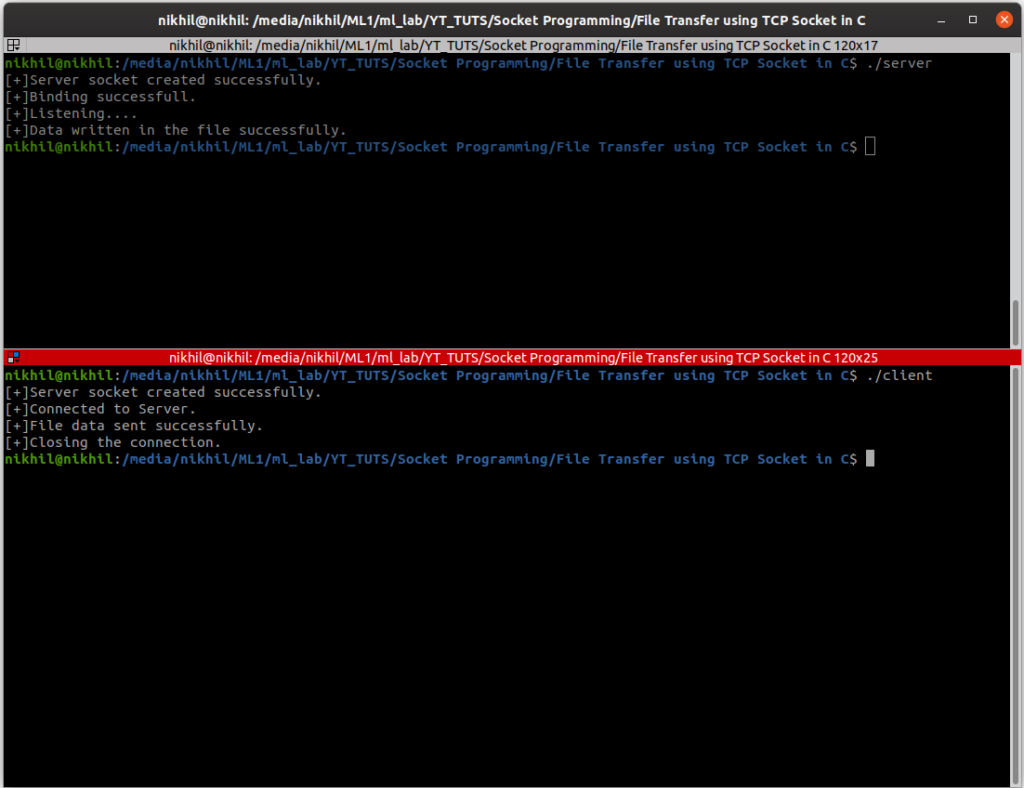
Summary
In this tutorial, you learn to implement a simple TCP file transfer using a client-server program in the C programming language. If you still have some questions or queries?
Contact:
Hi Nikhil,
Can I follow the same method when I am coding a multiple client – server system?
You can use select() to cater multiple client request.
Client on requesting a file, the server should send it by asking for the file name in the terminal. How to do it?
Can u please help?
Please let know how can I send an image?