File transfer has been one of many tasks in socket programming. In this article, we will discuss how to transfer large files using TCP sockets in Python. TCP (Transmission Control Protocol) is a reliable and efficient data transmission protocol commonly used for file transfers over the internet. With Python’s in-built socket library, it is easy to implement a TCP socket for file transfer purposes. This article will provide a step-by-step guide on how to create a TCP server and client in Python and how to send large files using this protocol. Whether you are a beginner or an experienced Python programmer, this article is designed to help you understand how to implement this solution. So, let’s dive into the world of TCP sockets and large file transfers in Python!
What is TCP?
TCP stands for Transmission Control Protocol. It is a type of computer networking protocol that is used to send data between computers in a network. The main job of TCP is to ensure that data is transmitted accurately and efficiently from one computer to another, regardless of any network issues that might arise.
TCP is a protocol that helps computers send data to each other in a reliable and efficient way.
Imagine you are sending a letter to someone through the postal service. The postal service is like the internet and the letter is like the data you are trying to send. Just like you want to make sure your letter reaches its destination safely and without any errors, the TCP protocol ensures that the data you send over the internet reaches its destination accurately and without any errors.
Related Tutorials
Project Structure
The project is divided into two files:
- server.py
- client.py
- friends-final.txt
The client.py contains the code, used to read a text file and send its data to the server. While the server.py file receives the data sent by the client and save it to a text file.
The friends-final.txt is the text file containing the textual data which needs to be transferred.
File Transfer: SERVER
The server performs the following functions:
- Create a TCP socket.
- Bind the IP address and PORT to the server socket.
- Listening for the clients.
- Accept the connection from the client.
- Receive the filename and its total size from the client.
- Send a response (Filename and filesize received from the client) back to the client.
- Create a text file.
- Start receiving a fix-size text data from the client.
- Write (save) the data into the text file.
- Send a response message back to the client.
- Repeat the step 8,9 and 10. Until the client send a blank data message. This means that the data transfer is completed.
- Close the text file.
- Close the connection.
import socket
from tqdm import tqdm
IP = socket.gethostbyname(socket.gethostname())
PORT = 4456
ADDR = (IP, PORT)
SIZE = 1024
FORMAT = "utf-8"
def main():
""" Creating a TCP server socket """
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind(ADDR)
server.listen()
print("[+] Listening...")
""" Accepting the connection from the client. """
conn, addr = server.accept()
print(f"[+] Client connected from {addr[0]}:{addr[1]}")
""" Receiving the filename and filesize from the client. """
data = conn.recv(SIZE).decode(FORMAT)
item = data.split("_")
FILENAME = item[0]
FILESIZE = int(item[1])
print("[+] Filename and filesize received from the client.")
conn.send("Filename and filesize received".encode(FORMAT))
""" Data transfer """
bar = tqdm(range(FILESIZE), f"Receiving {FILENAME}", unit="B", unit_scale=True, unit_divisor=SIZE)
with open(f"recv_{FILENAME}", "w") as f:
while True:
data = conn.recv(SIZE).decode(FORMAT)
if not data:
break
f.write(data)
conn.send("Data received.".encode(FORMAT))
bar.update(len(data))
""" Closing connection. """
conn.close()
server.close()
if __name__ == "__main__":
main()
File Transfer: CLIENT
The client performs the following functions:
- Create a TCP socket for the client.
- Connect to the server.
- Send the filename and total file size to the server.
- Receive the response (Filename and filesize received) from the server.
- Open the text file and start reading it.
- Send a fix-size text data to the server.
- Receive the response from the server.
- Repeat the 6 and 7. Until no data is left to be transferred.
- Close the file.
- Close the connection.
import os
import socket
from tqdm import tqdm
IP = socket.gethostbyname(socket.gethostname())
PORT = 4456
ADDR = (IP, PORT)
SIZE = 1024
FORMAT = "utf-8"
FILENAME = "friends-final.txt"
FILESIZE = os.path.getsize(FILENAME)
def main():
""" TCP socket and connecting to the server """
client = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client.connect(ADDR)
""" Sending the filename and filesize to the server. """
data = f"{FILENAME}_{FILESIZE}"
client.send(data.encode(FORMAT))
msg = client.recv(SIZE).decode(FORMAT)
print(f"SERVER: {msg}")
""" Data transfer. """
bar = tqdm(range(FILESIZE), f"Sending {FILENAME}", unit="B", unit_scale=True, unit_divisor=SIZE)
with open(FILENAME, "r") as f:
while True:
data = f.read(SIZE)
if not data:
break
client.send(data.encode(FORMAT))
msg = client.recv(SIZE).decode(FORMAT)
bar.update(len(data))
""" Closing the connection """
client.close()
if __name__ == "__main__":
main()
Output
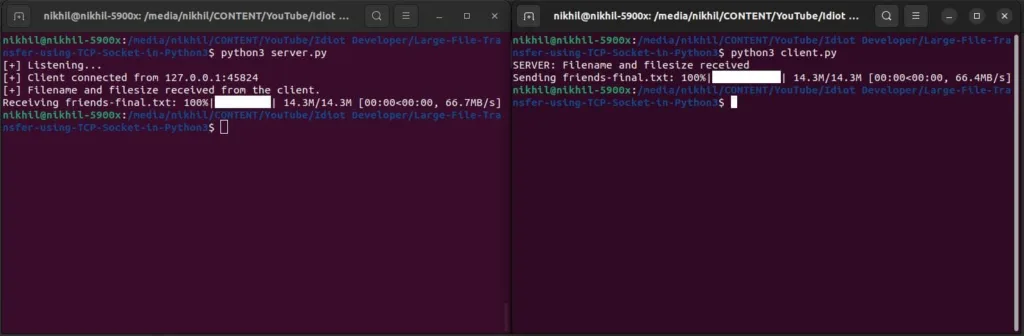
Summary
In this article, you have learned how to transfer a large text file in small chunks over TCP client-server connection in the python 3 programming language. Still, have some questions or queries? Just comment below. For more updates. Follow me.